Cartopy projection list#
PlateCarree#
- class cartopy.crs.PlateCarree(central_longitude=0.0, globe=None)[source]#
- Parameters:
proj4_params (iterable of key-value pairs) – The proj4 parameters required to define the desired CRS. The parameters should not describe the desired elliptic model, instead create an appropriate Globe instance. The
proj4_params
parameters will override any parameters that the Globe defines.globe (
Globe
instance, optional) – If omitted, the default Globe instance will be created. SeeGlobe
for details.
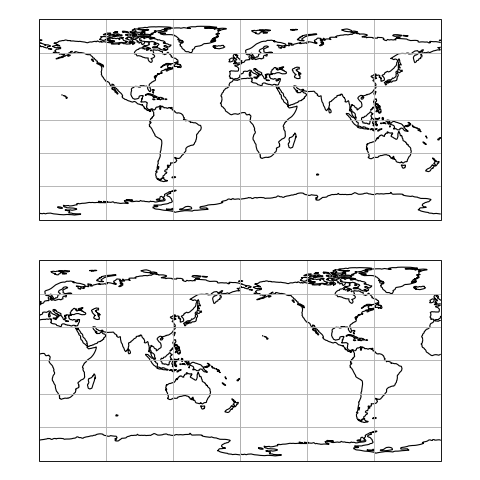
AlbersEqualArea#
- class cartopy.crs.AlbersEqualArea(central_longitude=0.0, central_latitude=0.0, false_easting=0.0, false_northing=0.0, standard_parallels=(20.0, 50.0), globe=None)[source]#
An Albers Equal Area projection
This projection is conic and equal-area, and is commonly used for maps of the conterminous United States.
- Parameters:
central_longitude (optional) – The central longitude. Defaults to 0.
central_latitude (optional) – The central latitude. Defaults to 0.
false_easting (optional) – X offset from planar origin in metres. Defaults to 0.
false_northing (optional) – Y offset from planar origin in metres. Defaults to 0.
standard_parallels (optional) – The one or two latitudes of correct scale. Defaults to (20, 50).
globe (optional) – A
cartopy.crs.Globe
. If omitted, a default globe is created.
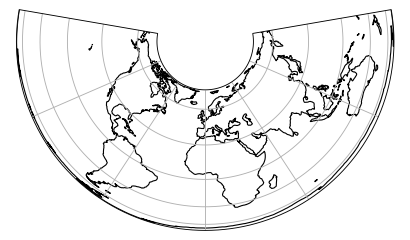
AzimuthalEquidistant#
- class cartopy.crs.AzimuthalEquidistant(central_longitude=0.0, central_latitude=0.0, false_easting=0.0, false_northing=0.0, globe=None)[source]#
An Azimuthal Equidistant projection
This projection provides accurate angles about and distances through the central position. Other angles, distances, or areas may be distorted.
- Parameters:
central_longitude (optional) – The true longitude of the central meridian in degrees. Defaults to 0.
central_latitude (optional) – The true latitude of the planar origin in degrees. Defaults to 0.
false_easting (optional) – X offset from the planar origin in metres. Defaults to 0.
false_northing (optional) – Y offset from the planar origin in metres. Defaults to 0.
globe (optional) – An instance of
cartopy.crs.Globe
. If omitted, a default globe is created.
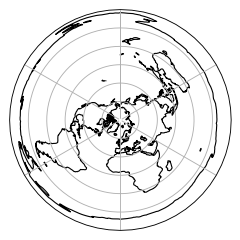
EquidistantConic#
- class cartopy.crs.EquidistantConic(central_longitude=0.0, central_latitude=0.0, false_easting=0.0, false_northing=0.0, standard_parallels=(20.0, 50.0), globe=None)[source]#
An Equidistant Conic projection.
This projection is conic and equidistant, and the scale is true along all meridians and along one or two specified standard parallels.
- Parameters:
central_longitude (optional) – The central longitude. Defaults to 0.
central_latitude (optional) – The true latitude of the planar origin in degrees. Defaults to 0.
false_easting (optional) – X offset from planar origin in metres. Defaults to 0.
false_northing (optional) – Y offset from planar origin in metres. Defaults to 0.
standard_parallels (optional) – The one or two latitudes of correct scale. Defaults to (20, 50).
globe (optional) – A
cartopy.crs.Globe
. If omitted, a default globe is created.
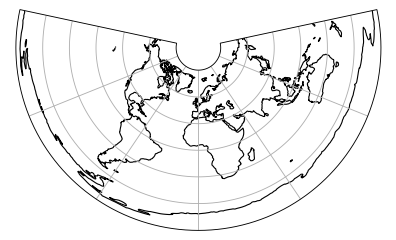
LambertConformal#
- class cartopy.crs.LambertConformal(central_longitude=-96.0, central_latitude=39.0, false_easting=0.0, false_northing=0.0, standard_parallels=(33, 45), globe=None, cutoff=-30)[source]#
A Lambert Conformal conic projection.
- Parameters:
central_longitude (optional) – The central longitude. Defaults to -96.
central_latitude (optional) – The central latitude. Defaults to 39.
false_easting (optional) – X offset from planar origin in metres. Defaults to 0.
false_northing (optional) – Y offset from planar origin in metres. Defaults to 0.
standard_parallels (optional) – Standard parallel latitude(s). Defaults to (33, 45).
globe (optional) – A
cartopy.crs.Globe
. If omitted, a default globe is created.cutoff (optional) – Latitude of map cutoff. The map extends to infinity opposite the central pole so we must cut off the map drawing before then. A value of 0 will draw half the globe. Defaults to -30.
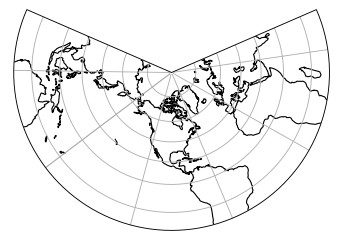
LambertCylindrical#
- class cartopy.crs.LambertCylindrical(central_longitude=0.0, globe=None)[source]#
- Parameters:
proj4_params (iterable of key-value pairs) – The proj4 parameters required to define the desired CRS. The parameters should not describe the desired elliptic model, instead create an appropriate Globe instance. The
proj4_params
parameters will override any parameters that the Globe defines.globe (
Globe
instance, optional) – If omitted, the default Globe instance will be created. SeeGlobe
for details.
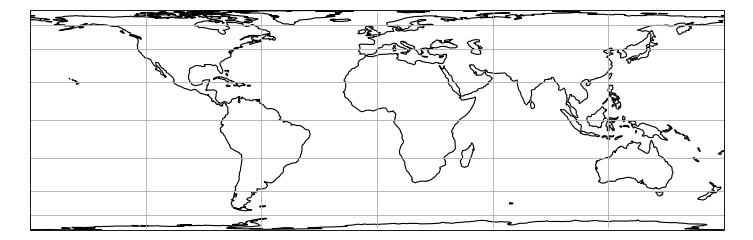
Mercator#
- class cartopy.crs.Mercator(central_longitude=0.0, min_latitude=-80.0, max_latitude=84.0, globe=None, latitude_true_scale=None, false_easting=0.0, false_northing=0.0, scale_factor=None)[source]#
A Mercator projection.
- Parameters:
central_longitude (optional) – The central longitude. Defaults to 0.
min_latitude (optional) – The maximum southerly extent of the projection. Defaults to -80 degrees.
max_latitude (optional) – The maximum northerly extent of the projection. Defaults to 84 degrees.
globe (A
cartopy.crs.Globe
, optional) – If omitted, a default globe is created.latitude_true_scale (optional) – The latitude where the scale is 1. Defaults to 0 degrees.
false_easting (optional) – X offset from the planar origin in metres. Defaults to 0.
false_northing (optional) – Y offset from the planar origin in metres. Defaults to 0.
scale_factor (optional) – Scale factor at natural origin. Defaults to unused.
Notes
Only one of
latitude_true_scale
andscale_factor
should be included.
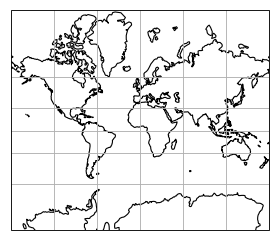
Miller#
- class cartopy.crs.Miller(central_longitude=0.0, globe=None)[source]#
- Parameters:
proj4_params (iterable of key-value pairs) – The proj4 parameters required to define the desired CRS. The parameters should not describe the desired elliptic model, instead create an appropriate Globe instance. The
proj4_params
parameters will override any parameters that the Globe defines.globe (
Globe
instance, optional) – If omitted, the default Globe instance will be created. SeeGlobe
for details.
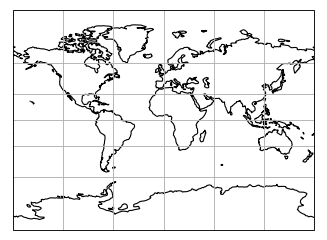
Mollweide#
- class cartopy.crs.Mollweide(central_longitude=0, globe=None, false_easting=None, false_northing=None)[source]#
A Mollweide projection.
This projection is pseudocylindrical, and equal area. Parallels are unequally-spaced straight lines, while meridians are elliptical arcs up to semicircles on the edges. Poles are points.
It is commonly used for world maps, or interrupted with several central meridians.
- Parameters:
central_longitude (float, optional) – The central longitude. Defaults to 0.
false_easting (float, optional) – X offset from planar origin in metres. Defaults to 0.
false_northing (float, optional) – Y offset from planar origin in metres. Defaults to 0.
globe (
cartopy.crs.Globe
, optional) –If omitted, a default globe is created.
Note
This projection does not handle elliptical globes.
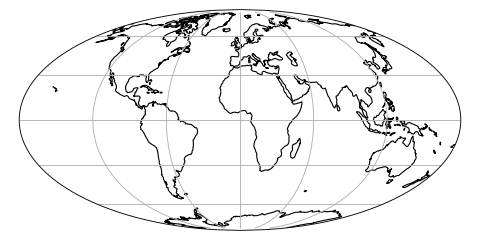
ObliqueMercator#
- class cartopy.crs.ObliqueMercator(central_longitude=0.0, central_latitude=0.0, false_easting=0.0, false_northing=0.0, scale_factor=1.0, azimuth=0.0, globe=None)[source]#
An Oblique Mercator projection.
- Parameters:
central_longitude (optional) – The true longitude of the central meridian in degrees. Defaults to 0.
central_latitude (optional) – The true latitude of the planar origin in degrees. Defaults to 0.
false_easting (optional) – X offset from the planar origin in metres. Defaults to 0.
false_northing (optional) – Y offset from the planar origin in metres. Defaults to 0.
scale_factor (optional) – Scale factor at the central meridian. Defaults to 1.
azimuth (optional) – Azimuth of centerline clockwise from north at the center point of the centre line. Defaults to 0.
globe (optional) – An instance of
cartopy.crs.Globe
. If omitted, a default globe is created.
Notes
The ‘Rotated Mercator’ projection can be achieved using Oblique Mercator with
azimuth
=90
.
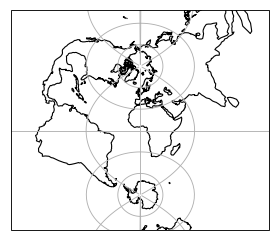
Orthographic#
- class cartopy.crs.Orthographic(central_longitude=0.0, central_latitude=0.0, globe=None)[source]#
- Parameters:
proj4_params (iterable of key-value pairs) – The proj4 parameters required to define the desired CRS. The parameters should not describe the desired elliptic model, instead create an appropriate Globe instance. The
proj4_params
parameters will override any parameters that the Globe defines.globe (
Globe
instance, optional) – If omitted, the default Globe instance will be created. SeeGlobe
for details.
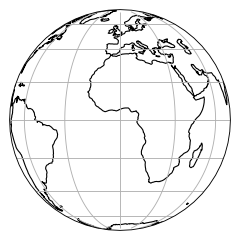
Robinson#
- class cartopy.crs.Robinson(central_longitude=0, globe=None, false_easting=None, false_northing=None)[source]#
A Robinson projection.
This projection is pseudocylindrical, and a compromise that is neither equal-area nor conformal. Parallels are unequally-spaced straight lines, and meridians are curved lines of no particular form.
It is commonly used for “visually-appealing” world maps.
- Parameters:
central_longitude (float, optional) – The central longitude. Defaults to 0.
false_easting (float, optional) – X offset from planar origin in metres. Defaults to 0.
false_northing (float, optional) – Y offset from planar origin in metres. Defaults to 0.
globe (
cartopy.crs.Globe
, optional) –If omitted, a default globe is created.
Note
This projection does not handle elliptical globes.
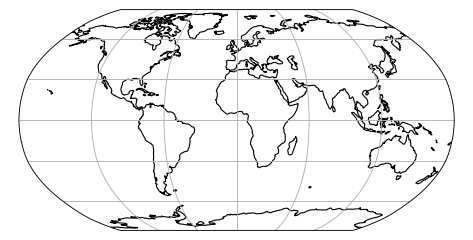
Sinusoidal#
- class cartopy.crs.Sinusoidal(central_longitude=0.0, false_easting=0.0, false_northing=0.0, globe=None)[source]#
A Sinusoidal projection.
This projection is equal-area.
- Parameters:
central_longitude (optional) – The central longitude. Defaults to 0.
false_easting (optional) – X offset from planar origin in metres. Defaults to 0.
false_northing (optional) – Y offset from planar origin in metres. Defaults to 0.
globe (optional) – A
cartopy.crs.Globe
. If omitted, a default globe is created.
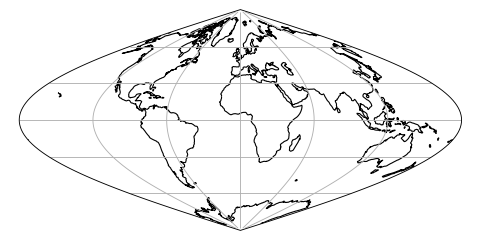
Stereographic#
- class cartopy.crs.Stereographic(central_latitude=0.0, central_longitude=0.0, false_easting=0.0, false_northing=0.0, true_scale_latitude=None, scale_factor=None, globe=None)[source]#
- Parameters:
proj4_params (iterable of key-value pairs) – The proj4 parameters required to define the desired CRS. The parameters should not describe the desired elliptic model, instead create an appropriate Globe instance. The
proj4_params
parameters will override any parameters that the Globe defines.globe (
Globe
instance, optional) – If omitted, the default Globe instance will be created. SeeGlobe
for details.
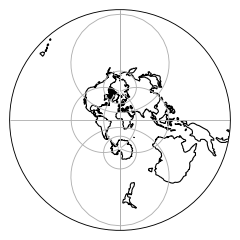
TransverseMercator#
- class cartopy.crs.TransverseMercator(central_longitude=0.0, central_latitude=0.0, false_easting=0.0, false_northing=0.0, scale_factor=1.0, globe=None, approx=False)[source]#
A Transverse Mercator projection.
- Parameters:
central_longitude (optional) – The true longitude of the central meridian in degrees. Defaults to 0.
central_latitude (optional) – The true latitude of the planar origin in degrees. Defaults to 0.
false_easting (optional) – X offset from the planar origin in metres. Defaults to 0.
false_northing (optional) – Y offset from the planar origin in metres. Defaults to 0.
scale_factor (optional) – Scale factor at the central meridian. Defaults to 1.
globe (optional) – An instance of
cartopy.crs.Globe
. If omitted, a default globe is created.approx (optional) – Whether to use Proj’s approximate projection (True), or the new Extended Transverse Mercator code (False). Defaults to True, but will change to False in the next release.
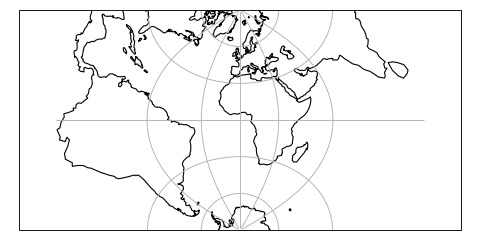
UTM#
- class cartopy.crs.UTM(zone, southern_hemisphere=False, globe=None)[source]#
Universal Transverse Mercator projection.
- Parameters:
zone – The numeric zone of the UTM required.
southern_hemisphere (optional) – Set to True if the zone is in the southern hemisphere. Defaults to False.
globe (optional) – An instance of
cartopy.crs.Globe
. If omitted, a default globe is created.
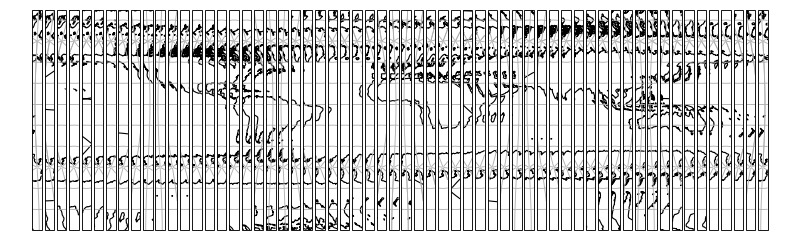
InterruptedGoodeHomolosine#
- class cartopy.crs.InterruptedGoodeHomolosine(central_longitude=0, globe=None, emphasis='land')[source]#
Composite equal-area projection emphasizing either land or ocean features.
- Original Reference:
Goode, J. P., 1925: The Homolosine Projection: A new device for portraying the Earth’s surface entire. Annals of the Association of American Geographers, 15:3, 119-125, DOI: 10.1080/00045602509356949
A central_longitude value of -160 is recommended for the oceanic view.
- Parameters:
central_longitude (float, optional) – The central longitude, by default 0
globe (
cartopy.crs.Globe
, optional) – If omitted, a default Globe object is created, by default Noneemphasis (str, optional) – Options ‘land’ and ‘ocean’ are available, by default ‘land’
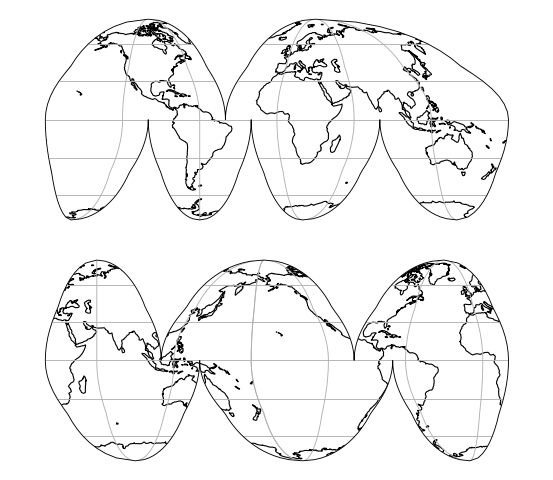
RotatedPole#
- class cartopy.crs.RotatedPole(pole_longitude=0.0, pole_latitude=90.0, central_rotated_longitude=0.0, globe=None)[source]#
A rotated latitude/longitude projected coordinate system with cylindrical topology and projected distance.
Coordinates are measured in projection metres.
The class uses proj to perform an ob_tran operation, using the pole_longitude to set a lon_0 then performing two rotations based on pole_latitude and central_rotated_longitude. This is equivalent to setting the new pole to a location defined by the pole_latitude and pole_longitude values in the GeogCRS defined by globe, then rotating this new CRS about it’s pole using the central_rotated_longitude value.
- Parameters:
pole_longitude (optional) – Pole longitude position, in unrotated degrees. Defaults to 0.
pole_latitude (optional) – Pole latitude position, in unrotated degrees. Defaults to 0.
central_rotated_longitude (optional) – Longitude rotation about the new pole, in degrees. Defaults to 0.
globe (optional) – An optional
cartopy.crs.Globe
. Defaults to a “WGS84” datum.
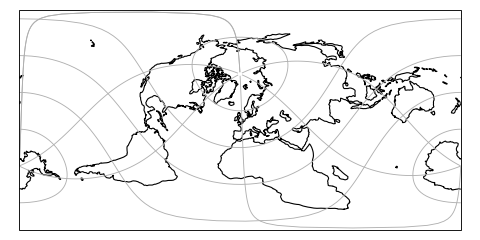
OSGB#
- class cartopy.crs.OSGB(approx=False)[source]#
- Parameters:
central_longitude (optional) – The true longitude of the central meridian in degrees. Defaults to 0.
central_latitude (optional) – The true latitude of the planar origin in degrees. Defaults to 0.
false_easting (optional) – X offset from the planar origin in metres. Defaults to 0.
false_northing (optional) – Y offset from the planar origin in metres. Defaults to 0.
scale_factor (optional) – Scale factor at the central meridian. Defaults to 1.
globe (optional) – An instance of
cartopy.crs.Globe
. If omitted, a default globe is created.approx (optional) – Whether to use Proj’s approximate projection (True), or the new Extended Transverse Mercator code (False). Defaults to True, but will change to False in the next release.
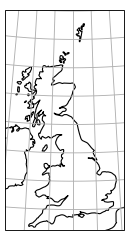
LambertZoneII#
- class cartopy.crs.LambertZoneII[source]#
Lambert zone II (extended) projection (https://epsg.io/27572), a legacy projection that covers hexagonal France and Corsica.
- Parameters:
proj4_params (iterable of key-value pairs) – The proj4 parameters required to define the desired CRS. The parameters should not describe the desired elliptic model, instead create an appropriate Globe instance. The
proj4_params
parameters will override any parameters that the Globe defines.globe (
Globe
instance, optional) – If omitted, the default Globe instance will be created. SeeGlobe
for details.
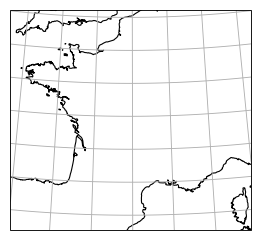
EuroPP#
- class cartopy.crs.EuroPP[source]#
UTM Zone 32 projection for EuroPP domain.
Ellipsoid is International 1924, Datum is ED50.
- Parameters:
zone – The numeric zone of the UTM required.
southern_hemisphere (optional) – Set to True if the zone is in the southern hemisphere. Defaults to False.
globe (optional) – An instance of
cartopy.crs.Globe
. If omitted, a default globe is created.
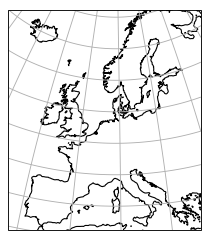
Geostationary#
- class cartopy.crs.Geostationary(central_longitude=0.0, satellite_height=35785831, false_easting=0, false_northing=0, globe=None, sweep_axis='y')[source]#
A view appropriate for satellites in Geostationary Earth orbit.
Perspective view looking directly down from above a point on the equator.
In this projection, the projected coordinates are scanning angles measured from the satellite looking directly downward, multiplied by the height of the satellite.
- Parameters:
central_longitude (float, optional) – The central longitude. Defaults to 0.
satellite_height (float, optional) – The height of the satellite. Defaults to 35785831 metres (true geostationary orbit).
false_easting – X offset from planar origin in metres. Defaults to 0.
false_northing – Y offset from planar origin in metres. Defaults to 0.
globe (
cartopy.crs.Globe
, optional) – If omitted, a default globe is created.sweep_axis ('x' or 'y', optional. Defaults to 'y'.) – Controls which axis is scanned first, and thus which angle is applied first. The default is appropriate for Meteosat, while ‘x’ should be used for GOES.
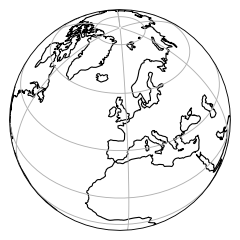
NearsidePerspective#
- class cartopy.crs.NearsidePerspective(central_longitude=0.0, central_latitude=0.0, satellite_height=35785831, false_easting=0, false_northing=0, globe=None)[source]#
Perspective view looking directly down from above a point on the globe.
In this projection, the projected coordinates are x and y measured from the origin of a plane tangent to the Earth directly below the perspective point (e.g. a satellite).
- Parameters:
central_longitude (float, optional) – The central longitude. Defaults to 0.
central_latitude (float, optional) – The central latitude. Defaults to 0.
satellite_height (float, optional) – The height of the satellite. Defaults to 35785831 meters (true geostationary orbit).
false_easting – X offset from planar origin in metres. Defaults to 0.
false_northing – Y offset from planar origin in metres. Defaults to 0.
globe (
cartopy.crs.Globe
, optional) –If omitted, a default globe is created.
Note
This projection does not handle elliptical globes.
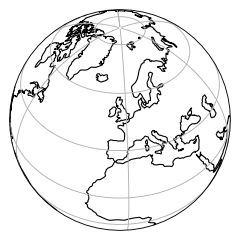
EckertI#
- class cartopy.crs.EckertI(central_longitude=0, false_easting=None, false_northing=None, globe=None)[source]#
An Eckert I projection.
This projection is pseudocylindrical, but not equal-area. Both meridians and parallels are straight lines. Its equal-area pair is
EckertII
.- Parameters:
central_longitude (float, optional) – The central longitude. Defaults to 0.
false_easting (float, optional) – X offset from planar origin in metres. Defaults to 0.
false_northing (float, optional) – Y offset from planar origin in metres. Defaults to 0.
globe (
cartopy.crs.Globe
, optional) –If omitted, a default globe is created.
Note
This projection does not handle elliptical globes.
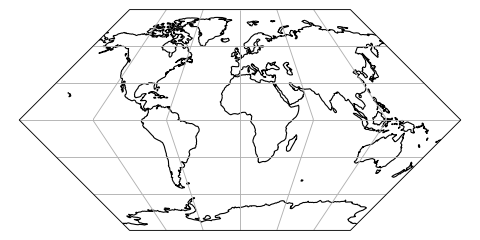
EckertII#
- class cartopy.crs.EckertII(central_longitude=0, false_easting=None, false_northing=None, globe=None)[source]#
An Eckert II projection.
This projection is pseudocylindrical, and equal-area. Both meridians and parallels are straight lines. Its non-equal-area pair with equally-spaced parallels is
EckertI
.- Parameters:
central_longitude (float, optional) – The central longitude. Defaults to 0.
false_easting (float, optional) – X offset from planar origin in metres. Defaults to 0.
false_northing (float, optional) – Y offset from planar origin in metres. Defaults to 0.
globe (
cartopy.crs.Globe
, optional) –If omitted, a default globe is created.
Note
This projection does not handle elliptical globes.
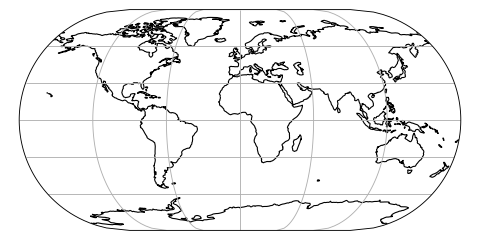
EckertIII#
- class cartopy.crs.EckertIII(central_longitude=0, false_easting=None, false_northing=None, globe=None)[source]#
An Eckert III projection.
This projection is pseudocylindrical, but not equal-area. Parallels are equally-spaced straight lines, while meridians are elliptical arcs up to semicircles on the edges. Its equal-area pair is
EckertIV
.- Parameters:
central_longitude (float, optional) – The central longitude. Defaults to 0.
false_easting (float, optional) – X offset from planar origin in metres. Defaults to 0.
false_northing (float, optional) – Y offset from planar origin in metres. Defaults to 0.
globe (
cartopy.crs.Globe
, optional) –If omitted, a default globe is created.
Note
This projection does not handle elliptical globes.
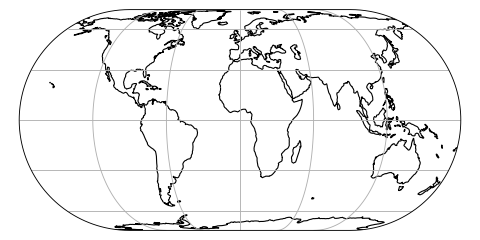
EckertIV#
- class cartopy.crs.EckertIV(central_longitude=0, false_easting=None, false_northing=None, globe=None)[source]#
An Eckert IV projection.
This projection is pseudocylindrical, and equal-area. Parallels are unequally-spaced straight lines, while meridians are elliptical arcs up to semicircles on the edges. Its non-equal-area pair with equally-spaced parallels is
EckertIII
.It is commonly used for world maps.
- Parameters:
central_longitude (float, optional) – The central longitude. Defaults to 0.
false_easting (float, optional) – X offset from planar origin in metres. Defaults to 0.
false_northing (float, optional) – Y offset from planar origin in metres. Defaults to 0.
globe (
cartopy.crs.Globe
, optional) –If omitted, a default globe is created.
Note
This projection does not handle elliptical globes.
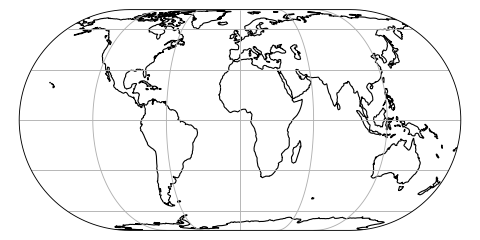
EckertV#
- class cartopy.crs.EckertV(central_longitude=0, false_easting=None, false_northing=None, globe=None)[source]#
An Eckert V projection.
This projection is pseudocylindrical, but not equal-area. Parallels are equally-spaced straight lines, while meridians are sinusoidal arcs. Its equal-area pair is
EckertVI
.- Parameters:
central_longitude (float, optional) – The central longitude. Defaults to 0.
false_easting (float, optional) – X offset from planar origin in metres. Defaults to 0.
false_northing (float, optional) – Y offset from planar origin in metres. Defaults to 0.
globe (
cartopy.crs.Globe
, optional) –If omitted, a default globe is created.
Note
This projection does not handle elliptical globes.
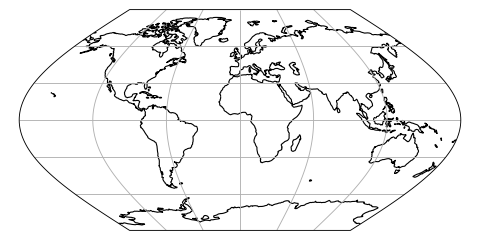
EckertVI#
- class cartopy.crs.EckertVI(central_longitude=0, false_easting=None, false_northing=None, globe=None)[source]#
An Eckert VI projection.
This projection is pseudocylindrical, and equal-area. Parallels are unequally-spaced straight lines, while meridians are sinusoidal arcs. Its non-equal-area pair with equally-spaced parallels is
EckertV
.It is commonly used for world maps.
- Parameters:
central_longitude (float, optional) – The central longitude. Defaults to 0.
false_easting (float, optional) – X offset from planar origin in metres. Defaults to 0.
false_northing (float, optional) – Y offset from planar origin in metres. Defaults to 0.
globe (
cartopy.crs.Globe
, optional) –If omitted, a default globe is created.
Note
This projection does not handle elliptical globes.
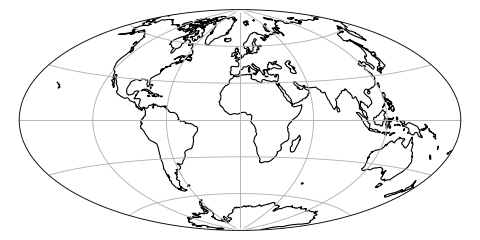
Aitoff#
- class cartopy.crs.Aitoff(central_longitude=0, false_easting=None, false_northing=None, globe=None)[source]#
An Aitoff projection.
This projection is a modified azimuthal equidistant projection, balancing shape and scale distortion. There are no standard lines and only the central point is free of distortion.
- Parameters:
central_longitude (float, optional) – The central longitude. Defaults to 0.
false_easting (float, optional) – X offset from planar origin in metres. Defaults to 0.
false_northing (float, optional) – Y offset from planar origin in metres. Defaults to 0.
globe (
cartopy.crs.Globe
, optional) –If omitted, a default globe is created.
Note
This projection does not handle elliptical globes.
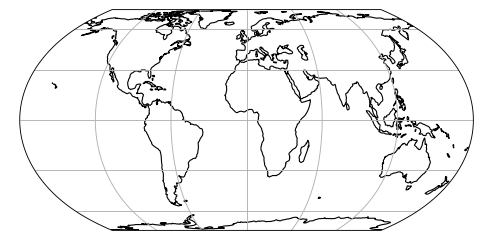
EqualEarth#
- class cartopy.crs.EqualEarth(central_longitude=0, false_easting=None, false_northing=None, globe=None)[source]#
An Equal Earth projection.
This projection is pseudocylindrical, and equal area. Parallels are unequally-spaced straight lines, while meridians are equally-spaced arcs.
It is intended for world maps.
Note
To use this projection, you must be using Proj 5.2.0 or newer.
References
Bojan Šavrič, Tom Patterson & Bernhard Jenny (2018) The Equal Earth map projection, International Journal of Geographical Information Science, DOI: 10.1080/13658816.2018.1504949
- Parameters:
central_longitude (float, optional) – The central longitude. Defaults to 0.
false_easting (float, optional) – X offset from planar origin in metres. Defaults to 0.
false_northing (float, optional) – Y offset from planar origin in metres. Defaults to 0.
globe (
cartopy.crs.Globe
, optional) – If omitted, a default globe is created.
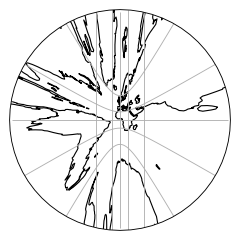
Gnomonic#
- class cartopy.crs.Gnomonic(central_latitude=0.0, central_longitude=0.0, globe=None)[source]#
- Parameters:
proj4_params (iterable of key-value pairs) – The proj4 parameters required to define the desired CRS. The parameters should not describe the desired elliptic model, instead create an appropriate Globe instance. The
proj4_params
parameters will override any parameters that the Globe defines.globe (
Globe
instance, optional) – If omitted, the default Globe instance will be created. SeeGlobe
for details.
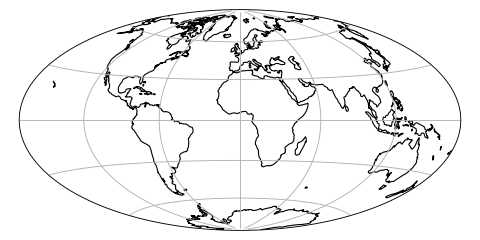
Hammer#
- class cartopy.crs.Hammer(central_longitude=0, false_easting=None, false_northing=None, globe=None)[source]#
A Hammer projection.
This projection is a modified
LambertAzimuthalEqualArea
projection, similar toAitoff
, and intended to reduce distortion in the outer meridians compared toMollweide
. There are no standard lines and only the central point is free of distortion.- Parameters:
central_longitude (float, optional) – The central longitude. Defaults to 0.
false_easting (float, optional) – X offset from planar origin in metres. Defaults to 0.
false_northing (float, optional) – Y offset from planar origin in metres. Defaults to 0.
globe (
cartopy.crs.Globe
, optional) –If omitted, a default globe is created.
Note
This projection does not handle elliptical globes.
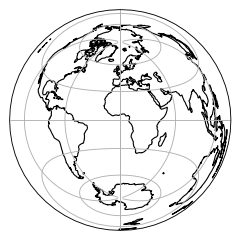
LambertAzimuthalEqualArea#
- class cartopy.crs.LambertAzimuthalEqualArea(central_longitude=0.0, central_latitude=0.0, false_easting=0.0, false_northing=0.0, globe=None)[source]#
A Lambert Azimuthal Equal-Area projection.
- Parameters:
central_longitude (optional) – The central longitude. Defaults to 0.
central_latitude (optional) – The central latitude. Defaults to 0.
false_easting (optional) – X offset from planar origin in metres. Defaults to 0.
false_northing (optional) – Y offset from planar origin in metres. Defaults to 0.
globe (optional) – A
cartopy.crs.Globe
. If omitted, a default globe is created.
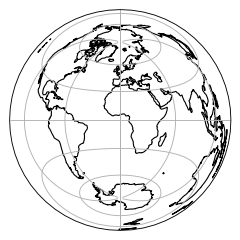
NorthPolarStereo#
- class cartopy.crs.NorthPolarStereo(central_longitude=0.0, true_scale_latitude=None, globe=None)[source]#
- Parameters:
proj4_params (iterable of key-value pairs) – The proj4 parameters required to define the desired CRS. The parameters should not describe the desired elliptic model, instead create an appropriate Globe instance. The
proj4_params
parameters will override any parameters that the Globe defines.globe (
Globe
instance, optional) – If omitted, the default Globe instance will be created. SeeGlobe
for details.
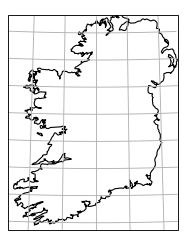
OSNI#
- class cartopy.crs.OSNI(approx=False)[source]#
- Parameters:
central_longitude (optional) – The true longitude of the central meridian in degrees. Defaults to 0.
central_latitude (optional) – The true latitude of the planar origin in degrees. Defaults to 0.
false_easting (optional) – X offset from the planar origin in metres. Defaults to 0.
false_northing (optional) – Y offset from the planar origin in metres. Defaults to 0.
scale_factor (optional) – Scale factor at the central meridian. Defaults to 1.
globe (optional) – An instance of
cartopy.crs.Globe
. If omitted, a default globe is created.approx (optional) – Whether to use Proj’s approximate projection (True), or the new Extended Transverse Mercator code (False). Defaults to True, but will change to False in the next release.
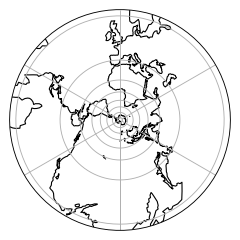
SouthPolarStereo#
- class cartopy.crs.SouthPolarStereo(central_longitude=0.0, true_scale_latitude=None, globe=None)[source]#
- Parameters:
proj4_params (iterable of key-value pairs) – The proj4 parameters required to define the desired CRS. The parameters should not describe the desired elliptic model, instead create an appropriate Globe instance. The
proj4_params
parameters will override any parameters that the Globe defines.globe (
Globe
instance, optional) – If omitted, the default Globe instance will be created. SeeGlobe
for details.
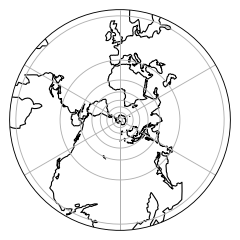